Hello everyone! Welcome to my third blog entry! In this blog entry, I will be documenting my experience in Arduino Programming, where I experimented with input and output devices to facilitate different purposes. A blog overview is provided below:
There are 4 tasks that will be explained in this blog: 1) Input devices: a. Interface a potentiometer analog input to maker UNO board and measure/show its signal in serial monitor Arduino IDE. b. Interface a LDR to maker UNO board and measure/show its signal in serial monitor Arduino IDE 2) Output devices: a. Interface 3 LEDs (Red, Yellow, Green) to maker UNO board and program it to perform something (fade or flash etc) b. Include the pushbutton on the MakerUno board to start/stop part 2.a. above
3) For each of the tasks, I will describe: 1. The program/code that I have used and explanation of the code. The code is in writable format (not an image). 2. The sources/references that I used to write the code/program. 3. The problems I encountered and how I fixed them. 4. The evidence that the code/program worked in the form of video of the executed program/code.
4) I will describe: My Learning reflection on the overall Arduino programming activities. |
Input devices: Interface a potentiometer analog input to maker UNO board and measure/show its signal in serial monitor Arduino IDE.
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int sensorPin = A0; int ledPin = 13; int sensorValue = 0; void setup () { pinMode (ledPin, OUTPUT); } void loop () { sensorValue = analogRead (sensorPin); digitalWrite (ledPin, HIGH); delay (sensorValue); digitalWrite (ledPin, LOW); delay (sensorValue); } | The sensorPin is initialized to be A0. The ledPin is initialized to be PIN 13. The sensorValue is initialised to be 0. Under the void setup section,
Under the void loop section lies the main code.
|
2. Below are the hyperlink to the sources/references that I used to write the code/program.
(from ESP Brightspace)
3. Below are the problems I have encountered and how I fixed them.
Initially, my setup did not work out and I was very distressed, until I realised that I had placed the black wire on the positive terminal on the breadboard instead of the negative terminal.
Also, my LED did not light up during my first try. After asking for help from peers, I reaslied that the positive and negative terminals of my LED were swapped.
However, after swapping them, my LED was really dim. To try to solve this problem, I experimented with different resistors, and even using the resistor with the lowest resistance did not result in my led being brighter. However, after I redid the setup from scratch and with a different LED, it worked.
4. Below is the short video as the evidence that the code/program works.
Input devices: Interface a LDR to maker UNO board and measure/show its signal in serial monitor Arduino IDE:
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int LDR = A0; int ledPin = 13; int LDRvalue = 0; void setup () { pinMode (ledPin, OUTPUT); } void loop () { LDRvalue = analogRead (LDR); if (LDRvalue > 950) digitalWrite (ledPin, HIGH); else digitalWrite (ledPin, LOW); } | PIN 13 is established as the LED pin and A0 is established as the LDR pin. The LDRvalue is initialised to be 0. Under the void setup section,
Under the void loop section lies the main code.
|
2. Below are the hyperlink to the sources/references that I used to write the code/program.
(from ESP Brightspace)
3. Below are the problems I have encountered and how I fixed them.
During my first attempt, I had forgotten to prove that when the LDR receives lesser light, L13 on the Arduino board will light up.
Hence, after analysing the code, I realised that LDRvalue > 600 is too low a value as the lighting in my environment gives a LDRvalue of around 900 and above, causing L13 on the arduino board to light up all the time, even when I did not cover the sensor. Hence, I made adjustments to the code and changed the LDRvalue to be > 950 instead. This then allowed L13 to not light up under normal lighting conditions and light up when i cover the sensor to decrease the light the LDR senses.
4. Below is the short video as the evidence that the code/program works.
Output devices: Interface 3 LEDs (Red, Yellow, Green) to maker UNO board and program it to perform something (fade or flash etc)
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int animationSpeed = 0; void setup () { pinMode (13, OUTPUT); pinMode (12, OUTPUT); pinMode (11, OUTPUT); } void loop () { animationSpeed = 400; digitalWrite (13, HIGH); delay (animationSpeed); // Wait for animationSpeed millisecond(s) digitalWrite (13, LOW); delay (animationSpeed); // Wait for animationSpeed millisecond(s) digitalWrite (12, HIGH); delay (animationSpeed); // Wait for animationSpeed millisecond(s) digitalWrite (12, LOW); delay (animationSpeed); // Wait for animationSpeed millisecond(s) digitalWrite (11, HIGH); delay (animationSpeed); // Wait for animationSpeed millisecond(s) digitalWrite (11, LOW); delay (animationSpeed); // Wait for animationSpeed millisecond(s) } | The animationSpeed is initialised to be 0. Under the void setup section,
Under the void loop section lies the main code.
Firstly, PIN 13 is ON, and after a specified delay time, PIN 13 is OFF. Next, after a specified delay time, PIN 12 is ON. After another specified delay time, PIN 12 is OFF. Next, after a specified delay time, PIN 11 is ON. After another specified delay time, PIN 11 is OFF. Lastly, after a specified delay time, the procedure restarts and continues the loop. |
2. Below are the hyperlink to the sources/references that I used to write the code/program.
(from Autodesk Tinkercad on YouTube)
3. Below are the problems I have encountered and how I fixed them.
Initially, all my LEDs were rather dim. After troubleshooting for a while, I realised that the ratings of the resistors I used were too high. To solve this problem, I used a resistor of a lower rating and my LEDs eventually became brighter.
4. Below is the short video as the evidence that the code/program work.
Output devices: Include pushbutton to start/stop the previous task
Instead of just including a pushbutton to start/stop the previous task, I decided to improvise a little and add two more LEDs to mimic a traffic light mechanism between vehicles and pedestrians.
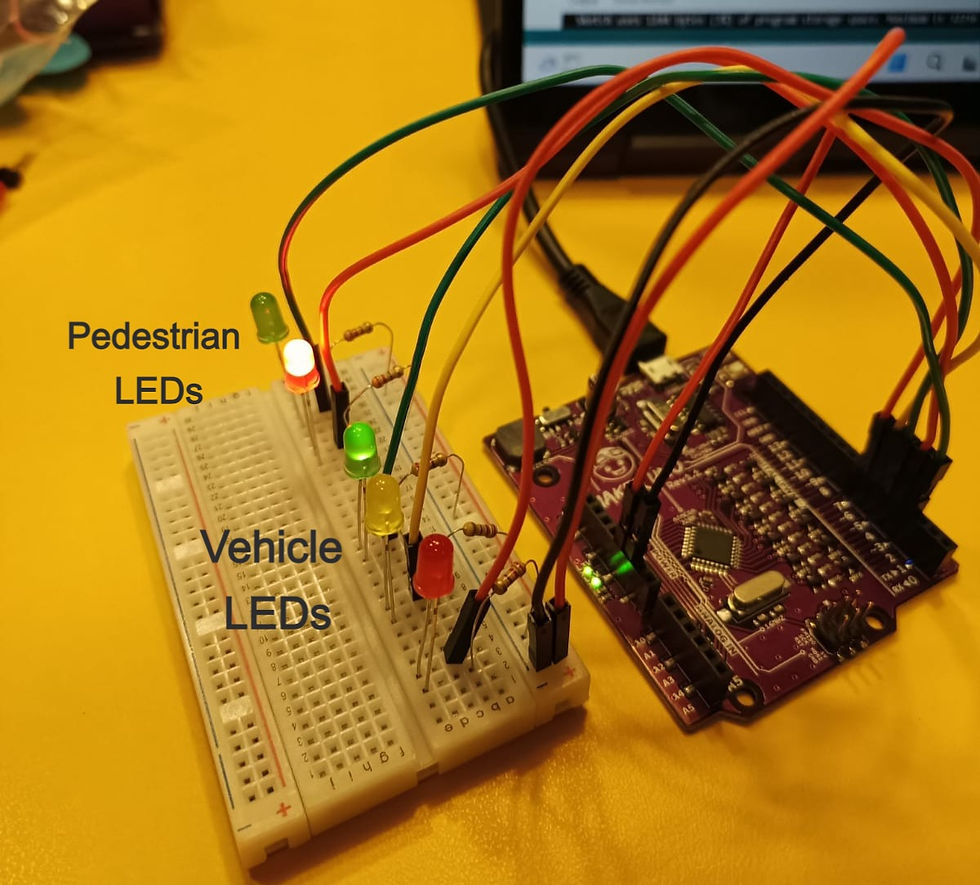
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
const int greenLedVehicle = 5; const int yellowLedVehicle = 6; const int redLedVehicle = 7; const int greenLedPedestrian = 3; const int redLedPedestrian = 4; const int pushButton = 2; void setup () { pinMode (greenLedVehicle, OUTPUT); pinMode (yellowLedVehicle, OUTPUT); pinMode (redLedVehicle, OUTPUT); pinMode (greenLedPedestrian, OUTPUT); pinMode (redLedPedestrian, OUTPUT); pinMode (pushButton, INPUT_PULLUP); digitalWrite (greenLedVehicle, HIGH); digitalWrite (redLedPedestrian, HIGH); } void loop () { if (digitalRead (pushButton) == LOW) { digitalWrite (greenLedVehicle, LOW); digitalWrite (yellowLedVehicle, HIGH); delay (2000); digitalWrite (yellowLedVehicle, LOW); digitalWrite (redLedVehicle, HIGH); delay (1000); digitalWrite (redLedPedestrian, LOW); digitalWrite (greenLedPedestrian, HIGH); delay (5000); digitalWrite (greenLedPedestrian, LOW); digitalWrite (redLedPedestrian, HIGH); delay (1000); digitalWrite (redLedVehicle, LOW); digitalWrite (greenLedVehicle, HIGH); } } | The declaration and initialization of variables was completed in the first section.
Under the void setup section,
Under the void loop section lies the main code.
When the button is pressed, Firstly, the green vehicle LED is OFF and the yellow vehicle LED will be ON. After a delay of 2 seconds, the yellow vehicle LED will be OFF and the red vehicle LED will be ON. This signals that vehicles are to come to a stop to allow pedestrians to cross the road. After a delay of 1 second, the red pedestrian LED will be OFF and the green pedestrian LED will be ON. This signals that pedestrians may cross the road. After a delay of 5 seconds, the green pedestrian LED is OFF and the red pedestrian LED is ON. This signals for pedestrians to stop crossing the road. After a delay of 1 second, the red vehicle LED is OFF and the green vehicle LED is ON. This signals that vehicles may continue driving forward. Another push of the push button will repeat the sequence above again. |
2. Below are the hyperlink to the sources/references that I used to write the code/program.
(from ESP Brightspace)
3. Below are the problems I have encountered and how I fixed them.
Surprisingly, completing this task was much smoother sailing as compared to the previous tasks, as I have already gotten used and conditioned to the operation of the Arduino UNO board.
4. Below is the short video as the evidence that the code/program works.
Learning Reflection
To be honest, learning about Arduino programming was the last thing on my mind when it comes to my course. Due to that, I was rather taken aback when I had seen it in the module overview and feared it, as I have heard from my peers from other courses that coding and programming is a challenging task. Hence, when we had started our first few lessons on Arduino programming, I was rather stressed out as the learning materials provided were rather lengthy and they were packed with information that I have never seen before, let alone understand. However, after being provided with the task to experiment with inputs and outputs, and eventually explaining the code used to program them, I grew more familiarised with Arduino programming as I started to understand each code for it. This made me feel more comfortable with it. Also, the learning package provided was a huge help as it had explained majority of the code commands, allowing me to understand codes better. I am thankful for such an informative learning package. Not to forget, other than the coding part of Arduino programming, the usage of the Arduino UNO board and the other components had also stressed me out as I had never dealt with such intricate items. I also had found understanding the importance of the placement of components on the Arduino UNO board and breadboard. However, after completing this task of experimenting with inputs and outputs, although I am still not very experienced with it, I am content to mention that I have definitely gotten more confident and well-versed with using the Arduino UNO board.
I was able to observe my improvement in Arduino Programming as during a practical session where my groupmate Joel and I were tasked to utilise a servo to flap a cardboard unicorn's wings, we managed to do it much easier due to our improved understanding of the coding commands. However, our unicorn's wings could not flap as much as the other groups' could, as our rubber band mechanism to secure the unicorn's wings to the servo was too stiff. Nevertheless, the session was fulfilling as instead of just flapping the unicorn's wings, we managed to make it flap along to a tune from the animated film: My Neighbour Totoro. The video below shows our completed setup.
Lastly, although learning about Arduino programming was tedious as it was a highly experimental process since I had to redo the codes and Arduino UNO board setup multiple times, I feel fulfilled as it was an interesting experience and it will definitely serve me good in my project design and development in the upcoming section of this module, and eventually my Capstone Project in Year 3.
Thank you everyone, for reading my blog entry and following me along my experience with Arduino programming, and I hope to see you again in my next blog entry! :-)
Comentários